Setting up your shell script
Last section we did some simple shell scripting. These shell scripts contained multiple commands and the script could be executed with bash. In this section, we go through some of the basics of shell scripts. Before getting started, however, you should know that when writing shell scripts in text editors, it's important that it supports bash. For example, running Sublime on the Windows OS will cause errors. If you're using Vim editor, you should have no problems.
Unix Command | Acronym translation | Description |
---|---|---|
which <COMMAND> | - | Shows the full path to the command. |
read [OPTION] [input1] [input2] [input3] | - | Can be used to prompt user for input and save them as variables [input1]..[input3]. |
Shebang '#!'
When making a shell script, the file extension '.sh' lets other users know that the file is a shell script. However, this sort of file extension doesn't actually affect how your system interprets the file. You could write whatever you want as your file extension and it wouldn't matter. What's important is to give your file something called a 'shebang' (which are the symbols '#!') as the first line. This is followed by a space and file path to the interpreter you would like to use in your script. This interpreter could be a shell but it could also be a programming language interpreter. In one case (1 in figure 1), we want to use bash as our interpreter. But we can change the interpreter to python3 interpreter (2 in figure 1). This, however, results in a syntax error because the command echo 'Hello' is not python language.
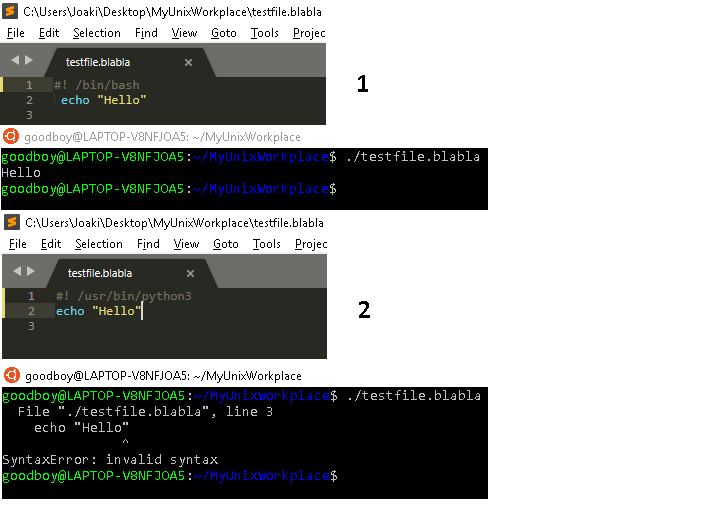
Also, bash is your default interpreter so if you were to delete the 'shebang' and just execute the file,
Prompt$ ./testfile.blabla
it would also work. However, if you were working on a server where bash wasn't the default interpreter, executing the file without shebangs might cause problems. Essentially, 'shebangs' ensure that your script is always executed with the intended interpreter, which in turn ensures that the file is executed properly.
Well almost always, as it's possible to forego 'shebangs' and use whatever interpreter you like from the command line. We've already been doing this earlier with bash.
Prompt$ bash <shell_script>
This would result in a file being interpreted by bash regardless of what has been specified in the file. This means that if you know which language a script is written in, you don't have to add a 'shebang'. But it's still a good practice. Just imagine a scenario, where you need to use multiple scripts written in different languages.
When making a 'shebang', you need to know where your interpreters are located. For shells, they'll typically be located in the directory /bin. You can check which shells are available on your system by looking in the file <shells>, Prompt$ cat /etc/shells
You can always check where commands/programs, such as interpreters, are located with the which command,
Prompt$ which [COMMAND]
which outputs the path to the specified Unix command. On a side note, it's worth knowing that when which and commands in general are executed, the shell will locate the command with the closest absolute path. This means that if you were to have two duplicate programs, it is the one with the closest absolute path that is used.
Comments
In shell scripting, you should make comments that explain your shell script. This can be done by entering the symbol '#' at the start of line, which results in the following text on that line, to be disregarded by your interpreter. So if you were to type,
# Comment for my shell script.
in some line in your shell script, your interpreter won't regard it as bash script.
Variables
When writing a shell script, you might want to define variables in your code. There are user and system variables. In figure 2, both types of variables are shown. A user variable like var1 can be assigned the value, 1, with the syntax,
var1=1
Values can be extracted by with a $ in front of the variable. So typing,
echo $var1
in a shell script will output '1'.
It's also possible to save the output of Unix commands as variables by using backtick symbols ``. This is shown in figure 2, with the command cal that displays a nice calendar. System variables, also called 'environment variables', are simply variables defined by your system. Examples of these are $SHELL, $BASH, $BASH_VERSION, $USER, $IFS, $HOME and (Internal field separator), etc. The environment variable, $SHELL, point two the shell that is used by default on your system. $BASH points to the execution path for bash (/bin/bash). Environment variables can change depending on which shell you're using, $BASH and $BASH_VERSION will for example not be valid when you're not using a BASH shell. The $HOME variable indicate where the home directory is located in your file system. You can list all of these commands by typing env' in your terminal.
Prompt$ env
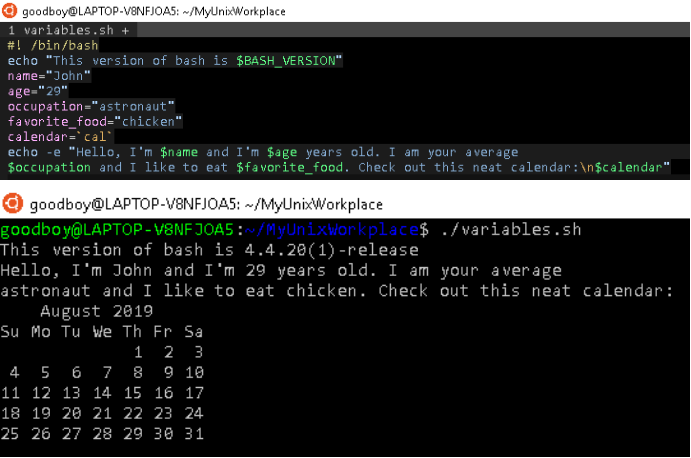
Variables are of course not limited to shell scripts, and you can also define variables in your terminal. The difference, however, is that the variables you set in your terminal, won't reset until your terminal session ends. When running a shell script, all variables that were set during the process, will reset after completion of the process.
Reading user input
Shell scripts can receive user input from the terminal, in the form of user arguments. It's quite simple to do as shown in figure 3.
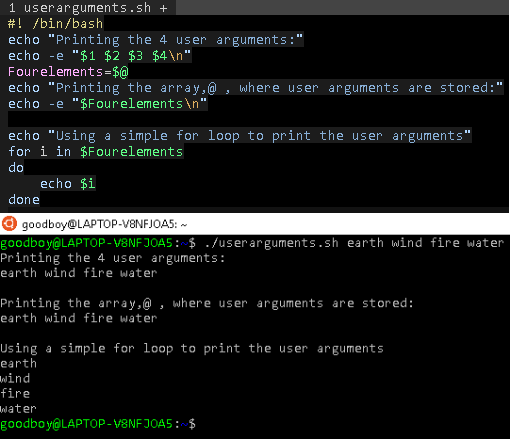
There's no limit to how many user arguments that can be passed to a file and they're saved as $1..$99 in the shell script. Furthermore, all user arguments are stored in the variable, @. So by typing,
Prompt$ echo $@
in your shell script, all user arguments will be outputted.
You can save all these user arguments to one variable,
var=$@
and extract user arguments separately using the syntax,
${var[i]}
which will extract the user argument at position i in var.
Shell scripts can also be made to prompt the user for input or multiple inputs with the command read, as shown in figure 4.
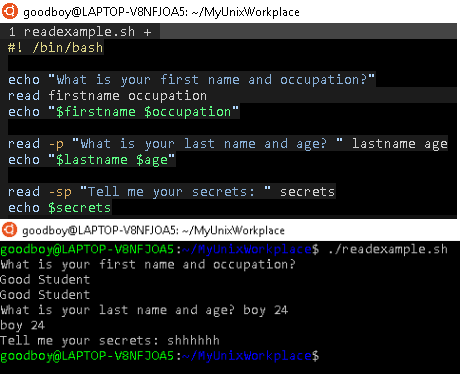
It's worth knowing that if you don't specify a variable with read, bash saves user input as a system variable called $REPLY. For example,
Prompt$ read && echo $REPLY
will output whatever you entered from your keyboard.
Exercise 1: User info file
Make a shell script that prompts the user for a username and password (make it stealthy). The information should be stored inside a file called <user_info.txt>. The username and password need to be stored neatly on separte lines likeso:
username:blabla password:blabla